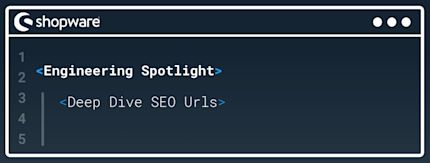
What are SEO URLs?
Typically, when you create a new controller, you might end up with a URL like this:
http://example.com/page/5
This URL is not user-friendly and provides no information about the page's content. SEO URLs, on the other hand, are designed to be more descriptive and meaningful. For example:
http://example.com/page/awesome-product-name
This URL is much more user-friendly and provides information about the page's content. It is also more likely to be clicked on by users, which can lead to higher click-through rates and better search engine rankings.
SEO URLs are generally essential, but especially for e-commerce websites. They help search engines understand the content of your pages and can improve your website's visibility in search results. In addition, SEO URLs can also enhance the user experience by making it easier for users to navigate your website and find the information they are looking for.
What are the typical ways of implementing SEO URLs?
There are several ways to implement SEO URLs in a web application. Some of the most common methods include
Using Slugs
Slugs are a common way to create SEO-friendly URLs. A slug is a URL-friendly string version, typically produced by converting the string to lowercase, replacing spaces with hyphens, and removing special characters. For example, the slug for "Awesome Product Name" would be "awesome-product-name".
So you would end up with a URL like this:
http://example.com/page/awesome-product-name
This looks exactly like the example above, but this system has some drawbacks. For example, if you would implement a controller for this, you would do something like this:
class PageController
{
#[Route('/page/{slug}')]
public function show(string $slug)
{
// Logic to retrieve the product by slug
}
}
So, the main drawback would be that we have a fixed prefix for the URL. Our URLs have to start with
/page
Using identifiers in the URL
Another common way to create SEO-friendly URLs is to use identifiers in the URL. This method involves using a unique identifier in the URL, such as a product ID or category ID. For example:
http://example.com/page/5/awesome-product-name
This URL includes the product ID (5) and the product name (awesome-product-name). This method is helpful, as we don't need to store slugs and look up by slug. Typically, you would implement this like this:
class PageController
{
#[Route('/page/{id}/{slug}')]
public function show(int $id, string $slug)
{
// Logic to retrieve the product by id
}
}
This method has the advantage of being more flexible, as we match only the id, and the other part is just a string, which we don’t care about. However, the page should have a canonical URL to avoid duplicate content. This is important for SEO, as search engines may penalize websites with duplicate content.
Dynamic URL routing
Dynamic URL routing is a more advanced method of creating SEO-friendly URLs. This method maps SEO URLs to specific controllers and actions in your application. For example, /awesome-product-page
links internally to /page/5
, but the user sees /awesome-product-page
. This method is more complex to implement, but it allows for greater flexibility and control over your URLs.
A database table representation would look like this:
SEO URL | Internal URL |
/awesome-product-page | /page/5 |
So, we can resolve the SEO URL to the internal URL and then use the internal URL to determine the controller.
A typical Symfony Application looks like this:

As the Router only knows our internal URLs, we need to resolve the SEO URL to the internal URL before implementing the logic for handling these routes.

With that approach, we get requests like /awesome-product-page
and resolve them to /page/5
, calling the router to resolve the controller and calling it. That's how Shopware is doing it internally.
This approach allows the customer to use whatever SEO URL they want.
How to generate SEO URLs to link to pages
As we have the table with the SEO URLs, we can just do a reverse search and get the SEO URL for a given internal URL. So we call the router to generate a URL (this would be the internal URL) and look in the Table to see if we have an SEO URL for that. If we have one, we can return the SEO URL, otherwise, we return the internal URL. Here is a pseudocode example of what this might look like:
public function generate(string $route, array $parameters): string
{
$originalUrl = $this->originalRouter->generate($route, $parameters);
// does an SQL query to fetch the seo url if possible
return $this->lookupByOriginalUrl($originalUrl);
}
This approach works. But when you call the Router 20x, it will also do 20x a SQL query.
A good approach could be to fetch all the pages we want to show on our page, collect the internal URLs, and then do a single query to fetch all SEO URLs for these internal URLs and attach the information to the page.
In Shopware, we chose a similar approach, but instead of doing it for all entities, we solved it generally for all entities. We have built our own seoUrl Twig function, which internally generates an internal URL and returns it with a specific marker. So, if the internal URL is /page/5
, the seoUrl
function would return 124c71d524604ccbad6042edce3ac799/page/5#
.
When the View has been rendered and the page is ready to be sent to the browser, we have a listener that searches the HTML for this marker, does one single query to fetch all SEO URLs for the internal URLs, and replaces the internal URL with the SEO URL.
This way, we are flexible, generate only the URLs we need, and have a single query to fetch all SEO URLs.
Conclusion
In this blog post, we have explored the world of SEO URLs and how they work in Shopware. We have discussed the different methods of implementing SEO URLs, including slugs, identifiers, and dynamic URL routing. We have also looked at how to generate SEO URLs to link to pages and how Shopware handles SEO URLs internally.