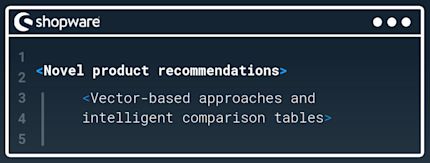
The possibilities of product recommendations in ecommerce are constantly evolving. While many standard solutions are based on static algorithms, innovative approaches such as vector-based recommendations and dynamic comparison tables can significantly improve the user experience and increase the conversion rate.
This blog post covers two modern approaches that developers can implement regardless of their platform:
Vector-based product recommendations: Recommendations that analyze semantic similarities between products.
Intelligent comparison tables: Dynamic comparisons of products with UX optimizations that only display relevant information.
1. Vector-based product recommendations
Vector-based product recommendations utilize semantic similarities between product features. Unlike traditional methods that use categories or purchase histories, vector-based systems calculate the proximity between products in the vector space. Products with similar attributes therefore appear more natural and relevant in the recommendations.
How it works
Each product is represented by a vector that contains numerical values for important attributes (e.g. color, material, price). Similarities between products are calculated using the distance between these vectors, e.g. cosine similarity.
function cosineSimilarity(array $vecA, array $vecB): float {
$dotProduct = array_sum(array_map(fn($a, $b) => $a * $b, $vecA, $vecB));
$magnitudeA = sqrt(array_sum(array_map(fn($a) => $a ** 2, $vecA)));
$magnitudeB = sqrt(array_sum(array_map(fn($b) => $b ** 2, $vecB)));
return ($magnitudeA * $magnitudeB) > 0 ? $dotProduct / ($magnitudeA * $magnitudeB) : 0.0;
}
$currentProductVector = [0.7, 0.2, 0.1];
$otherProducts = [
["name" => "product A", "vector" => [0.65, 0.25, 0.1]],
["name" => "product B", "vector" => [0.1, 0.8, 0.1]],
["name" => "product C", "vector" => [0.6, 0.15, 0.25]],
];
$recommendations = array_map(function ($product) use ($currentProductVector) {
$similarity = cosineSimilarity($currentProductVector, $product['vector']);
return ['name' => $product['name'], 'similarity' => $similarity];
}, $otherProducts);
usort($recommendations, fn($a, $b) => $b['similarity'] <=> $a['similarity']);
print_r($recommendations);
2. Intelligent comparison tables
Challenge: Dynamics and relevance in product selection
Comparison tables are helpful when products have similar properties. But what happens when products differ greatly? Nobody wants to compare a fridge with a laptop. To improve the user experience, comparison tables should be dynamically controlled by displaying only relevant products and attributes.
Solutions for a better UX:
Relevance filter for products: Comparison tables should only display products with similar attributes. This can be ensured by a category filter or attribute matching.
Dynamic attribute display: Tables should only display attributes that differ between products. This maintains clarity.
Fallback options: If no relevant products are found, another recommendation mechanism (e.g. ‘similar categories’) can take effect.
Here is an example of an intelligent comparison table with filter and dynamic options.
// Example products $products = [ ["name" => "product A", "price" => 99.99, "material" => "Leather", "category" => "Fashion", "warranty" => "2 years"], ["name" => "product B", "price" => 89.99, "material" => "Fabric", "category" => "Fashion", "warranty" => "1 years"], ["name" => "product C", "price" => 109.99, "material" => "Synthetic leather", "category" => "Fashion", "warranty" => "3 years"], ["name" => "product D", "price" => 499.99, "material" => "Metal", "category" => "Electronics", "warranty" => "2 years"], ];
// Filter: Only show products from the same category $currentCategory = "Fashion"; $filteredProducts = array_filter($products, fn($product) => $product['category'] === $currentCategory);
// Select dynamic attributes $attributes = ["price", "material", "warranty"]; $dynamicAttributes = array_keys(array_filter( array_reduce($filteredProducts, function ($carry, $product) use ($attributes) { foreach ($attributes as $attr) { $carry[$attr][$product[$attr]] = true; } return $carry; }, []), fn($values) => count($values) > 1 ));
// Generate table function generateDynamicComparisonTable(array $products, array $attributes): string { $table = "<table border='1' style='width:100%; text-align:left;'>"; $table .= "<tr><th>Produkt</th>"; foreach ($attributes as $attribute) { $table .= "<th>" . ucfirst($attribute) . "</th>"; } $table .= "</tr>";
foreach ($products as $product) { $table .= "<tr><td>{$product['name']}</td>"; foreach ($attributes as $attribute) { $table .= "<td>{$product[$attribute]}</td>"; } $table .= "</tr>"; } $table .= "</table>"; return $table; }
echo generateDynamicComparisonTable($filteredProducts, $dynamicAttributes);
Functionality:
Relevance filter: Only products with the same category are included in the table.
Dynamic attributes: Only attributes that differ between the products are displayed.
Fallback option: Products without a basis for comparison can alternatively be replaced by ‘better alternatives in the same category’.
Conclusion
With these two approaches, developers can:
Implement vector-based recommendations that are contextually and semantically more accurate than classic methods.
Create intelligent comparison tables that dynamically adjust relevant products and attributes to optimize the user experience.
These concepts are system-agnostic and offer inspiring approaches for modern product recommendations that can be easily integrated into existing systems.
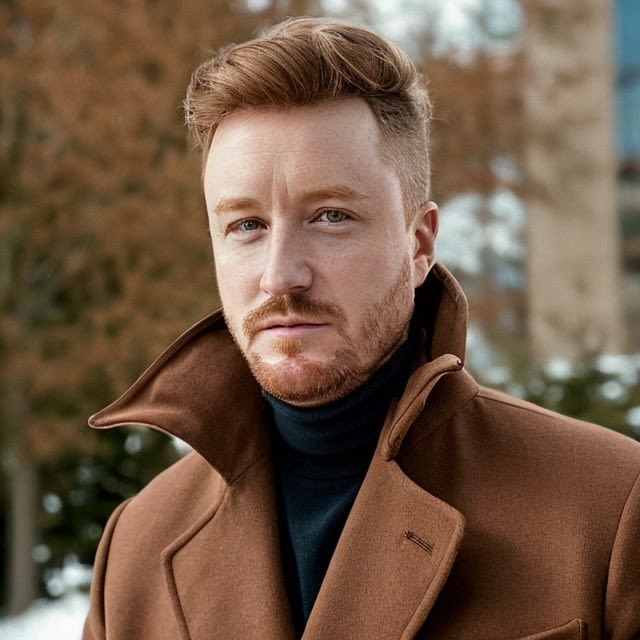